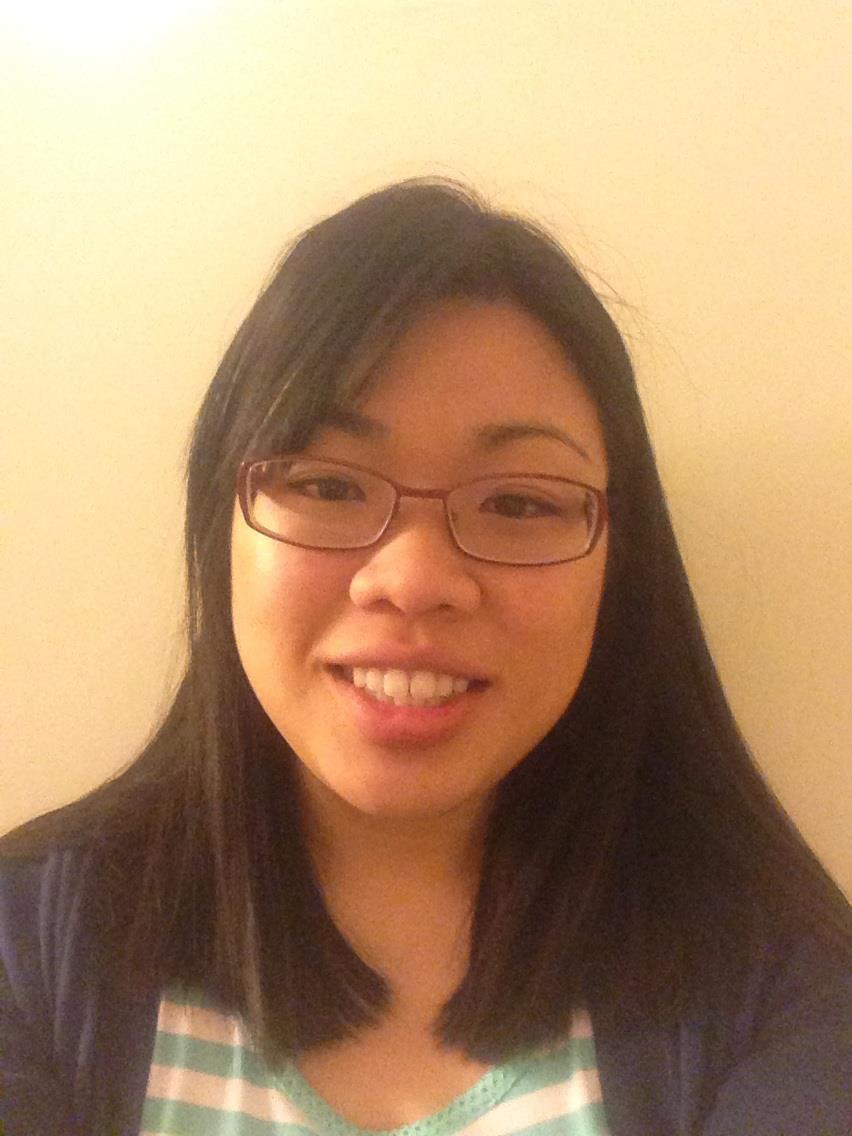
How To Make New Terminals Have Diffferent Background Colors
I often have multiple terminal windows open at once and want them to have different backgrounds so that I can more easily differentiate between them. This is how I made it happen with iTerm2 and zsh
.
Iterm2 allows us to change the background color on the command line using escape codes described here.
echo -e "\033]1337;SetColors=bg=522\a"
The -e
option expands the escape codes. 522
is the specified color in RGB format with three hex digits. You can read more about what the escape codes mean here. Because I knew I wasn’t going to remember how that command worked, I put that command in a function set-iterm2-background
with named variables to document how it works.
I then created function cycle-background
that randomly sets the background color to one in the RGB range 222
-999
to set limits on brightness. Smaller numbers represent brighter colors and larger, darker. 000
is white and fff
is black.
######################################################
### appearance #######################################
######################################################
function set-iterm2-background() {
echo "setting iterm2 background color $1"
# The following formats are accepted:
# RGB (three hex digits, like fff)
# RRGGBB (six hex digits, like f0f0f0)
# cs:RGB (like RGB but cs gives a color space)
# cs:RRGGBB (like RRGGBB but cs gives a color space)
# https://askubuntu.com/questions/831971/what-type-of-sequences-are-escape-sequences-starting-with-033
## iterm2 has escape codes that let you config on the cli
## https://iterm2.com/documentation-escape-codes.html
ESC_CHAR_IN_OCTAL="\033"
INTRO_OPERATING_SYSTEM_COMMAND="${ESC_CHAR_IN_OCTAL}]"
INTRO_IT2="${INTRO_OPERATING_SYSTEM_COMMAND}1337"
BELL_ALERT_CHAR="\a"
background="bg"
echo -e "${INTRO_IT2};SetColors=${background}=${1}${BELL_ALERT_CHAR}"
}
function cycle-background() {
# echo -e for expand esc chars, behavior depends on terminal
let "MINIMUM = 222"
let "MAXIMUM = 777"
RANGE=$((MAXIMUM - MINIMUM))
set-iterm2-background $(( $RANGE * $RANDOM / 32767 + $MINIMUM))
}
cycle-background
Note about zsh
Initially, I tried setting ZSH_THEME="random"
in my .zshrc
, but found that none of the pre-installed themes configured the background color. They just all assumed a dark background. It is possible to change the background color using zsh
. I just decided against that route.