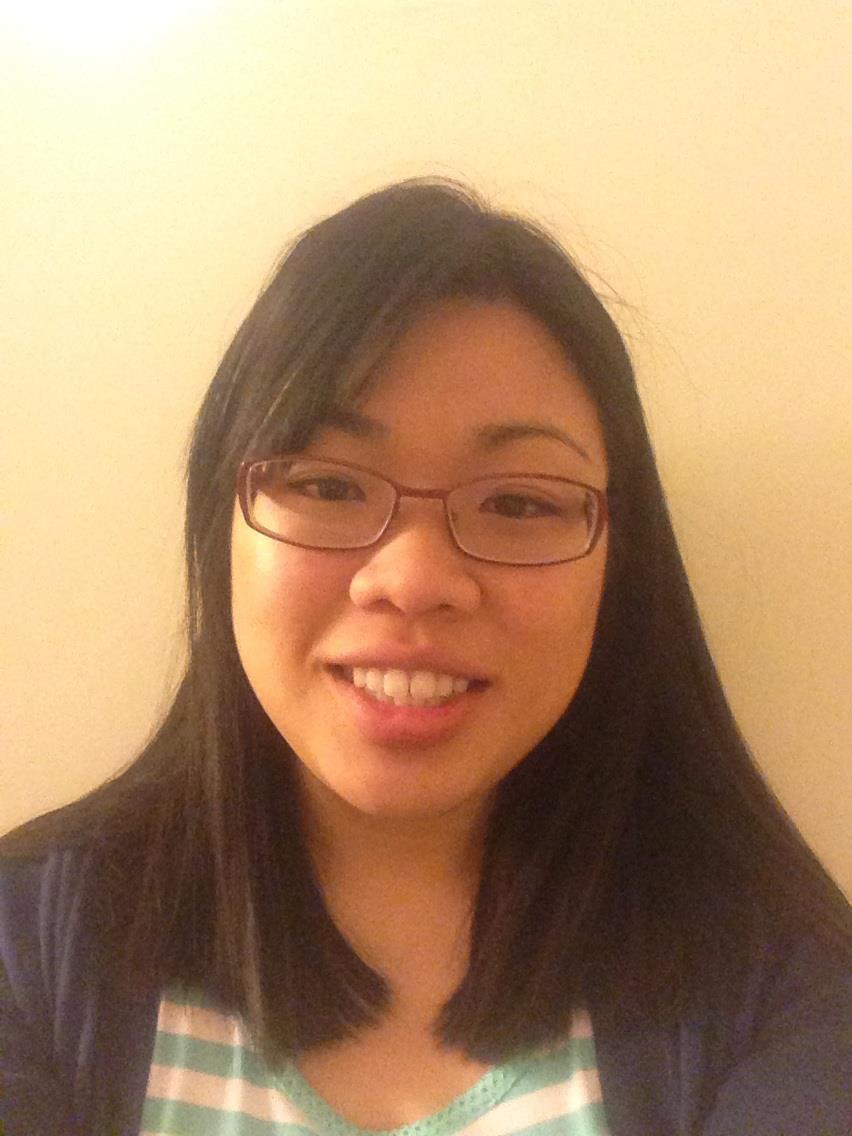
How to Manually Print Double-Sided
I have an old printer that can’t print double-sided anymore. I want to use up all my toner for that printer before buying a new printer. I wrote code so that I can manually print double-sided. This has saved me a couple hundred dollars.
Shortcomings
It’s not perfect. In certain cases when you want to print multiple pages on one side of a sheet of paper, you may need to specifiy placeholder pages for blank sheets. For example, if you want to print 7 pages, two pages per side of a sheet of paper, then the directions are to first print pages (7, None, 3, 4)
. None
is a blank page, but we can’t tell specify blank pages. I tried, so I’ve just been usig a placeholder. If I’m lucky, my file already has a blank page somewhere.
Assumptions
Every printer is different. My printer printed on the top face of the sheets in the paper tray and placed the printed side face down. This code only works for printers with this configuration. The finished printed pages will be face down. I may update it for other configurations, and I’m also open to contributors.
Set start_p
and end_p
to the starting and ending page numbers of the pages you want to print respectively. Then set pages_per_side
to the number of pages you want per side of a sheet of paper. Then run the Python script. It will print out directions.
from collections import namedtuple
from itertools import chain as flatten
start_page = 5 #input starting page number
end_page = 7 #input ending page number
pages_per_side = 2
pp = list(range(start_page, end_page+1))
spelled_numbers = ['one', 'two', 'three', 'four',
'five', 'six', 'seven', 'eight',
'nine', 'ten', 'eleven', 'twelve']
pages_on_side = spelled_numbers[:pages_per_side]
Side = namedtuple('Side', pages_on_side, defaults=[None]*pages_per_side)
sides = [Side(*pp[i:i+pages_per_side]) for i in range(0, len(pp), pages_per_side)]
Sheet = namedtuple('Sheet', ['front', 'back'],
defaults=(Side(*[None]*pages_per_side),))
sheets = [Sheet(*sides[i:i+2]) for i in range(0, len(sides), 2)]
backs = [s.back for s in sheets[::-1]]
is_implied_blank = backs[0] == Side(None)
first_pass = backs[1:] if is_implied_blank else backs
second_pass = [s.front for s in sheets]
print("""Print the following page numbers in the first pass.
Replace None with placeholder pages. Ideally, we want the printer to print blank pages
where None should be, but I don't know how to do that. """)
print(", ".join((str(page_number) for page_number in flatten(*first_pass))))
print()
print("""When done finishing printing the first pass, place printed pages
in printer tray with the appropriate side facing up.
Print the following page numbers.""")
print(", ".join((str(page_number) for page_number in flatten(*second_pass))))